I’ve been mucking about with others’ Hydra examples since I saw the movies of it at max. Basically its a language based on C designed to perform image processing tasks. Eventually Hydra will be implemented into after effects, photoshop and a subset of the specs into the flash player.
Here are the results of my experiments:
—————————————————————
1. MASK
The following code takes two images, gets the grayscale of the mask and then the alpha of the base image to the grayscale.
kernel mask{void evaluatePixel(in image4 src,in image4 mask, out pixel4 dst)
{
float4 maskImage = sampleNearest(mask, outCoord());
float4 outputImage = sampleNearest(src, outCoord());
float grayscale = (maskImage.r + maskImage.g + maskImage.b) / 3.0;
outputImage.a = grayscale;
dst = outputImage;
}
}
The following two input images (the hello as the mask)
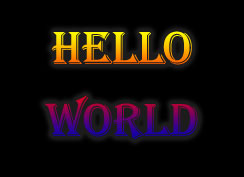
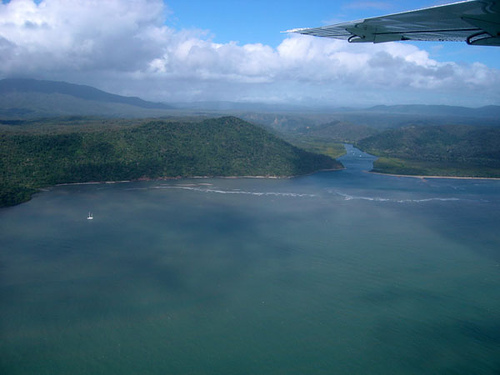
Produce:
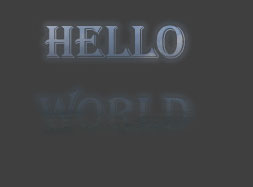
Grey is the default background colour so the image is transparent over that.
———————————————————–
2: WIGGLE
I also created a wiggle filter
kernel morph
{parameter float xangle<
minValue: 0.0;
maxValue:10.0;
defaultValue:5.0;
>;
parameter float yangle<
minValue: 0.0;
maxValue:10.0;
defaultValue:0.0;
>;
void evaluatePixel(in image4 src, out pixel4 dst)
{
float2 coord = outCoord();
float xcoord = coord.x - sin(coord.y)*xangle;
float ycoord = coord.y - sin(coord.x)*yangle;
dst = sampleNearest(src,float2(xcoord,ycoord));
}
}
This filter takes inputs of the wiggle size of the x and y and produces the following examples:
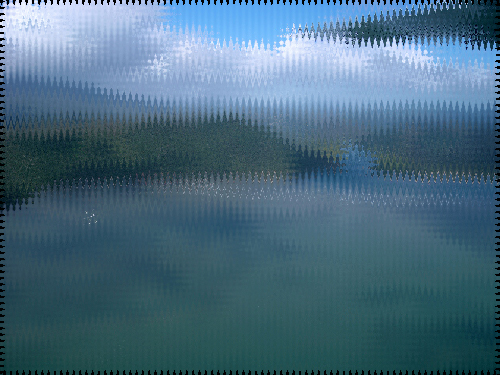
Wiggle on both the x and the y
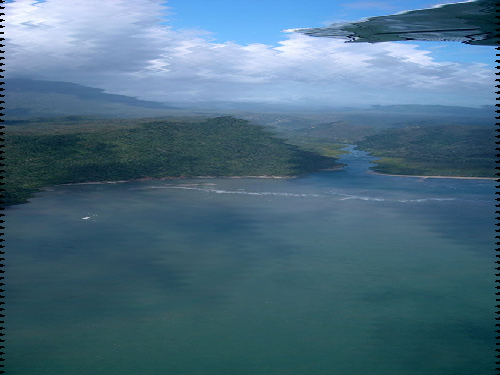
a wiggle just on the x
—————————————————————–
3. FLIP
And an flip filter. In the documentation there is a referenceFrame parameter to get the bounds of the input image. However after experimenting with it I couldn’t get it to work. I posted on the adobe forums and found out that it has not been implemented yet, so having a parameter with the width will have to do for now.
kernel flip
parameter float width< minValue: 100.0;
maxValue: 200.0;
defaultValue:500.0;
description: "Width of image";
>;
void evaluatePixel(in image4 src, out pixel4 dst)
{
float2 coord = outCoord();
float2 newcoord = float2(width-coord.x,coord.y);
dst = sampleNearest(src,newcoord);
}
}
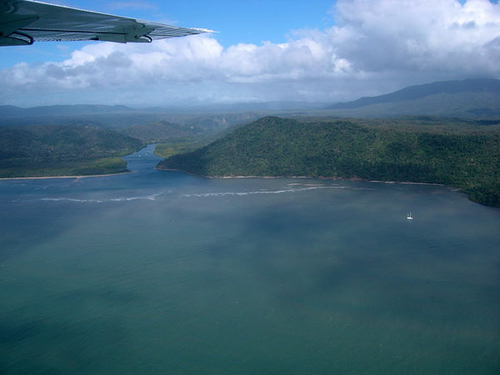